⚠️ Warning: this is an old article and may include information that’s out of date. ⚠️
Color keywords
Example usage:
// Example body { color: red; } // Generalization (not actual code) body { color: color_name; }
Because color names are easy to remember, they’re handy for getting quick results, especially while doing rapid prototyping.
W3C’s CSS1 recommendation first mentioned 16 color keywords that you can use: aqua, black, blue, fuchsia, gray, green, lime, maroon, navy, olive, purple, red, silver, teal, white, and yellow:
Color |
---|
aqua / cyan |
black |
blue |
fuchsia / magenta |
(color table from Wikipedia)
CSS2 officially mapped the colors to recommended hex values and also added the color orange. CSS3 removed orange, presumably because having 17 colors was aesthetically unpleasing in a binary world?
In addition to these 16 “official” colors, there’s even more unofficial color names you can use that appear to be supported in all the major browsers.
RGB values
Example usage:
// Example body { color: rgb(255,0,0); } // Generalization (not actual code) body { color: rgb(red [integer 0-255], green [integer 0-255], blue [integer 0-255]); }
For this section it’s very handy to know a bit about color theory, particularly these facts:
- all colors can be made from a combination of red, green, and blue (RGB) as the primary colors
- in light (additive color mixing), the absence of all color results in the color black
- in light (additive color mixing), the mixing of red, green, and blue in equal amounts results in the color white
With the rgb attribute, we specify the amount of each color we want with an integer from 0 to 255. Here we can see both black and white expressed as rgb values:
Black:
rgb(0,0,0)
White:
rgb(255,255,255)
Also note that if all three values are equal, there is no color that predominates and the result is a shade of gray.
Dark gray:
rgb(30,30,30)
A lighter shade of gray:
rgb(200,200,200)
And of course we can favor only one color, which in this case gives us a full red, with no green or blue:
rgb(255,0,0)
You may have guessed that we can also use percentage values to represent the same color:
rgb(100%, 0%, 0%)
RGBA values
// Example body { color: rgba(255,0,0,0.5); } // Generalization (not actual code) body { color: rgb(red [integer 0-255], green [integer 0-255], blue [integer 0-255], alpha transparency [float 0-1]); }
CSS3 introduced rgba, which is in every way similar to rgb, except for the addition of a fourth value, alpha opacity, which gives us control over the transparency of the color.
So the equivalent full red we have above would look like this (no transparency):
rgba(255, 0, 0, 1)
rgba(100%, 0%, 0%, 1)
And the same color, only now semitransparent:
rgba(255, 0, 0, 0.5)
rgba(100%, 0%, 0%, 0.5)
Hex values
Example usage:
// Example body { color: #ff0000; } // Generalization (not actual code) body { color: #rrggbb; }
Hex values are probably the most common out of all of the ways to represent colors in CSS. But I included them last – what gives? It seems to me that the rgb value should logically be explained first. The hex value is just a hex version (and thus slightly more confusing version) of expressing rgb values.
For this section you ought to know the basics of hexadecimal numbers. In particular, the fact that hexadecimals are base 16 and use letters a-f to represent numbers beyond our conventional base 10 range.
The hex value starts with a hash (#) and is followed by six numbers and/or letters. This is simply our three color values again: red, green, blue, with each color allowed two digits.
We express our solid red color like this:
#ff0000
As long as you can convert between decimal and hexadecimal (there’s a few free tools online), it’s relatively easy to convert between rgb and hex color values. Just keep in mind that the first 16 hex values are preceded by a zero (0 becomes 00, 1 becomes 01, 10 becomes 0a, 11 becomes 0b, etc.)
Shorthand hex
Example usage:
// Example body { color: #f00; } // Generalization (not actual code) body { color: #rgb; }
Last but not least, if each rgb value has a repeating value, you can simply omit the repeating value. In the case above each of our color values was repeating (red = ff, green = 00, blue = 00), so we simply drop the repeating digit from each color and cut three bytes from our code:
#f00
A note on web-safe colors
Web safe colors were a recommended subset of about 256 colors with which to design websites. The rationale for solely using them for web design was for rendering consistency, so even users with very limited displays could see the page as it was intended to be displayed.
The concept of a “web safe” color was becoming obsolete even in 1997 or so, when I started making my personal website on AOL. It’s safe to say that the vast majority of users today are now able to view webpages with at least 16 million colors (256*256*256).
But this doesn’t mean we shouldn’t be concerned about colors. Apart from pure aesthetics, in the area of accessibility it’s often mentioned that users with colorblindness find certain combinations of colors difficult to see. So keep this in the back of your head when you go crazy with those wild color schemes!
More info
Probably the best way to learn how to use these color values is to actually try them on your website, however you might also find the following links useful.
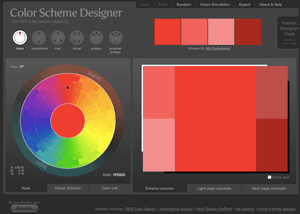
Color Scheme Designer is a nice tool for finding several colors that (theoretically) should go well together. It’s a nice place to go when building a website from the ground-up.
<p>
<a href="http://colorschemedesigner.com/">http://colorschemedesigner.com/</a>
</p>
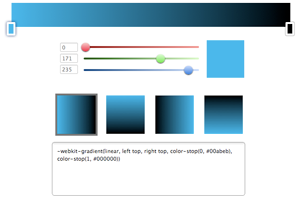
Jonathan Schlaepfer made a nice helper tool built in Mootols for creating webkit gradients. Gradients wasn’t the subject of this entry, but it’s helpful to see a nice visualization of rgb values corresponding to the hex values.
<p>
<a href="http://schlaeps.com/mootools/gradient-creator/">http://schlaeps.com/mootools/gradient-creator/</a>
</p>
Comments